This is just a sample that you may adapt to your needs, it uses B11:B20 as NewRange
:
Sub SortElseWhere()
Dim A As Range, NewRange As Range
Set A = Range("A1:A10")
Set NewRange = Range("B11:B20")
A.Copy NewRange
NewRange.Sort Key1:=NewRange(1, 1), Order1:=xlAscending, Header:=xlNo, _
OrderCustom:=1, MatchCase:=False, Orientation:=xlTopToBottom, _
DataOption1:=xlSortNormal
End Sub
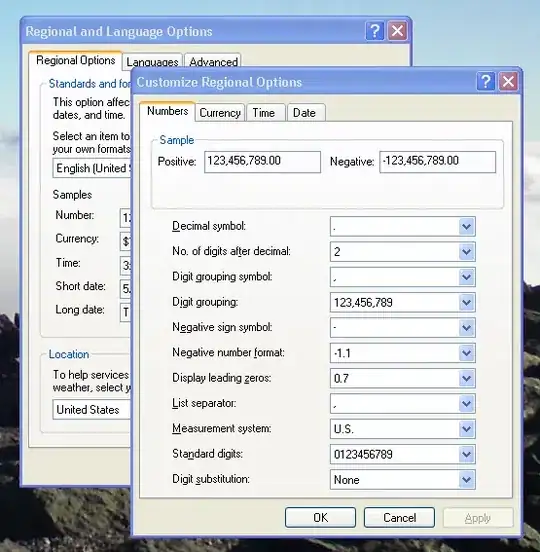
The original cells are not sorted, they are merely copied to another location which is sorted.
EDIT#1:
In this version, NewRange
is not a range of cells, but an internal array:
Sub SortElseWhere2()
Dim A As Range, NewRange(1 To 10) As Variant
Dim i As Long, strng As String
i = 1
Set A = Range("A1:A10")
For Each aa In A
NewRange(i) = aa
i = i + 1
Next aa
Call aSort(NewRange)
strng = Join(NewRange, " ")
MsgBox strng
End Sub
Public Sub aSort(ByRef InOut)
Dim i As Long, J As Long, Low As Long
Dim Hi As Long, Temp As Variant
Low = LBound(InOut)
Hi = UBound(InOut)
J = (Hi - Low + 1) \ 2
Do While J > 0
For i = Low To Hi - J
If InOut(i) > InOut(i + J) Then
Temp = InOut(i)
InOut(i) = InOut(i + J)
InOut(i + J) = Temp
End If
Next i
For i = Hi - J To Low Step -1
If InOut(i) > InOut(i + J) Then
Temp = InOut(i)
InOut(i) = InOut(i + J)
InOut(i + J) = Temp
End If
Next i
J = J \ 2
Loop
End Sub
