You can do it with two lines of code, with the help of pandas groupby
and plotly's barmode
attribute.
Plotly's bar chart has a specific attribute to control how to show the bars, it's called barmode
, quoting the API documentation:
barmode: str (default 'relative'
)
One of 'group'
, 'overlay'
or 'relative'
In 'relative'
mode,
bars are stacked above zero for positive values and below zero for
negative values. In 'overlay'
mode, bars are drawn on top of one
another. In 'group'
mode, bars are placed beside each other.
See the bar chart documentation for examples.
Now, for your example:
# import needed libraries
import pandas as pd
import plotly.express as px
# some dummy dataset
df = pd.DataFrame(
{
"Name": ["User1", "User1", "User1", "User2"],
"Defect severity": ["Medium", "Medium", "High", "High"],
}
)
You need to group by both Name
and Defect severity
columns, and then use the count
aggregating function (I recommend you take a look at this question)
df = df.groupby(by=["Name", "Defect severity"]).size().reset_index(name="counts")
The data now will look like the following:
|
Name |
Defect severity |
counts |
0 |
User1 |
High |
1 |
1 |
User1 |
Medium |
2 |
2 |
User2 |
High |
1 |
Finally, you can use plotly bar chart:
px.bar(data_frame=df, x="Name", y="counts", color="Defect severity", barmode="group")
The chart would be:
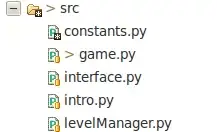
There you go! with only two lines of code, you got a nice grouped bar chart.