What caused this
Below is a simple explanation.
transform.position
is Vector3
.
Vector3
is a struct
.
Struct is a value type.
It's always good look in the Unity's Documentation to see which type Unity API is. This really matter when working in Unity.
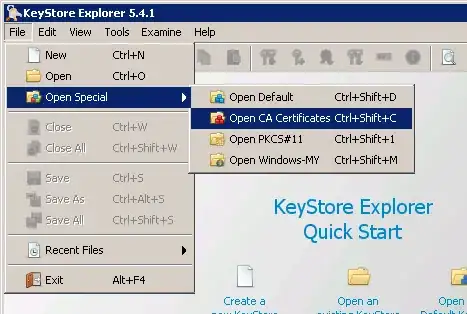
When you do:
vector3 playerPos = transform.position;
New struct
is created and values from transform.position;
is copied to playerPos
variable. Since the variable is value type and copied, performing System.Object.ReferenceEquals on it should return false
.
I want to create a vector3-type object referring to my player's
position so that I can use a shorter
In this case, you have to change the Vector3
to Transform
.
Transform myPos = null;
void Start()
{
myPos = transform;
}
then you can do:
myPos.position = newPos;
The reason you have to declare the position as Transform
instead of Vector3
is because Transform
is a class and a class stores the reference of the object.
class is a reference type.
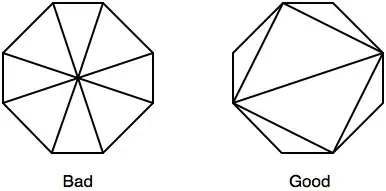
Debug.Log(System.Object.ReferenceEquals(myPos, transform));
should return true
because myPos
and transform
are both classes and the myPos
variable is referencing the transform
variable.
So, whenever you access your position with myPos.position;
, it will give you the newest position of your GameObject which is also (transform.position
) since the myPos
variable is a class
that has a reference of transform
which is also a class
.
Assuming that myPos
is struct
or declared as vector3 myPos
instead of Transform myPos
, then the myPos
variable would hold the copy value of transform.position
when it was assigned and will never return the latest position of your GameObject since it is a copy.
You can learn more about class
vs struct
here.
Finally System.Object.ReferenceEquals
says "ReferenceEquals" not "ValueEquals" so this is even self-explanatory. You use it on a class
/referece types not on structs
or value types.