Your boolean expression using ìnstanceof`is correct. I would suspect that the method that you use to fill the apps static field from AppointmentBook class is the source of the issue. That's the only logical explanation if debugging shows that every if statement is being skipped. I tried to reproduce some code similar to yours in order to test it and it is working fine.
Here is what I did
First an Appointment class:
public class Appointment {
}
Second an AppointmentBook class
import java.util.ArrayList;
import java.util.List;
public class AppointmentBook {
public static List<Appointment> apps = new ArrayList<Appointment>();
public AppointmentBook addAppointment(Appointment app) {
apps.add(app);
return this;
}
}
Third a OneTime class that extends Appointment (since you said Appointment is the superclass of OneTime)
public class OneTime extends Appointment {
public boolean occursOn(int month, int day, int year) {
if (day >= 15) {
return true;
} else {
return false;
}
}
}
As you can see I am using a trivial test case to return boolean results from the occursOn method (simply for test purpose)
And then I created the following test class. I fill the AppointmentBook apps with four Appointment instances, of which two are "instanceof" OneTime
public class AppointmentTest {
static int year = 2015;
static int month = 3;
static int day = 15;
public static void main(String[] args) {
AppointmentBook book = new AppointmentBook();
book.addAppointment(new Appointment())
.addAppointment(new OneTime())
.addAppointment(new Appointment())
.addAppointment(new OneTime());
for (Appointment item: AppointmentBook.apps) {
if (item instanceof OneTime) {
boolean checkOnce = ((OneTime)item).occursOn(month, day, year);
if (checkOnce == true) {
System.out.println("We have a checked OneTime instance...");
} else {
System.out.println("We have an unchecked OneTime instance...");
}
} else {
System.out.println("Not a OneTime instance...");
}
}
}
}
The result obtained is shown in the following image: it proves that your instanceof expression is correct and the issue most probably is related to the method that fills the apps field
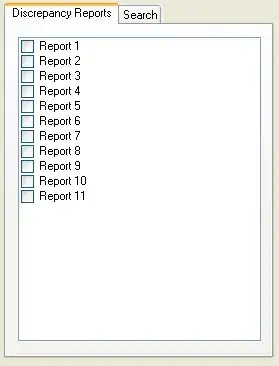