How do you use programming languages and scripting languages together?
Scripting languages ARE programming languages. You are likely thinking of the typical alternative to scripting/interpreted languages: Compiled languages.
I always hear of people using multiple languages in a program
Languages are sometimes mixed together in the source code because they can be. Some languages are built on older languages and have compilers that can work with the older version. Good old c should be available to you if you're programming in c++. Most c++ compilers also allow you to drop in assembly code. The reason to do this can range from a technical requirement (performance, etc) that one language can't fulfil on it's own, to the programmer simply feeling more comfortable solving a particular problem in that other language.
Othertimes languages that have no compiler (or interpreter) in common are used together to create a single program. This is thanks to a linker that can connect them together. DLL libraries are a good example of this. I don't care what a DLL library is written in so long as it works and publishes an API I can understand.
Sometimes different languages make different executables that aren't connected by a linker. But as long as they can run, and communicate with each other, they can work together. If you have an mp3 recorder written in java and a player written in c++ they can work together simply by agreeing on what an mp3 is.
... explain to me the difference between what I know as programming languages and scripts
The difference between compiled languages and interpreted languages, also known as scripts, is how they are run or executed. Any compiled language must be compiled before it can be run. Interpreted languages aren't. There are two surprising things to understand here: What compiled means and why it's needed. That is to say, why every language isn't interpreted.
Compiling a language involves many steps but the heart of it is turning a txt file into a binary file. This binary file will contain machine code. Machine code is the fundamental language your computer speaks. These are the famous 1's and 0's that everyone talks about but never sees. Hang on and I'll show them to you.
A text file is something humans can read with notepad (or VI, or TextEdit, or...) in fact if you're reading this you're reading a txt file right now.
A binary file is something that usually looks like martians wrote it when you open it in notepad. They look like this:
An executable program opened in a text editor
Most of this looks like nonsense. That's because it's not written in human language. This is machine language. This is what is easy for computer's to understand. Part of why it looks like nonsense is because notepad assumes it's a txt file and tries to present it as one. Only actual txt in the executable binary is readable by humans.
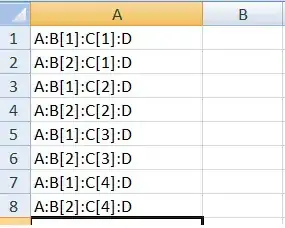
Same executable program opened in a hex editor
Here we can see the same file presented as a massive number shown in something called hexadecimal. We show binary files like this because showing them in binary takes more space.
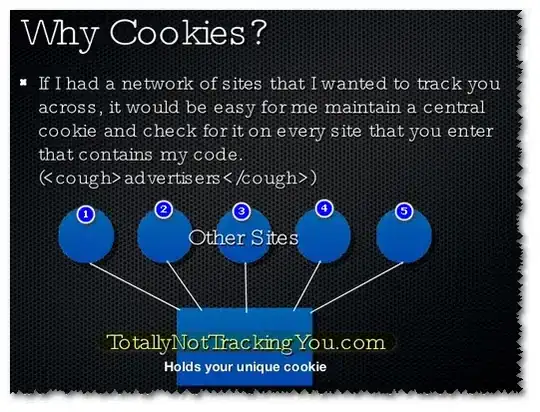
A small snippet of the huge number entered in a calculator in hex mode.
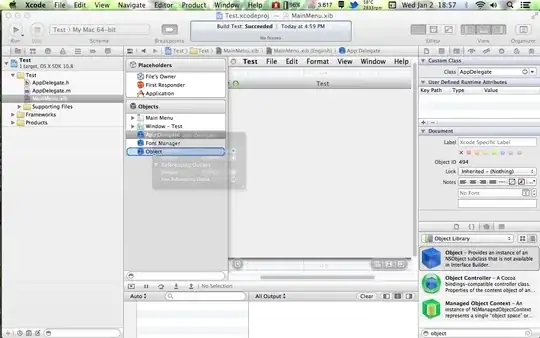
Same number as snippet but presented in binary. Here are your famous 1's and 0's.
If you look back at the Hex editor picture you'll see that it has a little something in it you can read, "This program cannot be run in DOS"
That is a string of text that has been embedded in the binary executable. And it's a big hint about what is going on. It turns out that txt files are binary files as well. Every file is a binary file in our binary computers. So what is the real difference? Encoding!
Encoding is how you code information. An ascii txt file uses numbers that range from 0-127. There are other txt file codes codes but ascii is a popular one. When you write a script or when you write source code for a compiled (or interpreted) language you're encoding information in ascii (or unicode or ...).
Compiling a language really means translating this txt file that into a machine code file. When that is run the computer isn't slowed down by having to do the translation again. The machine code file can also be made smaller since it they encode information more compactly and the compiler throws out what it doesn't need, like your variable names.
An interpreted language must do this same translation at the same time it's trying to run the program. That typically slows it down though modern interpreters and virtual machines like to brag about how they can do a few things faster because they have the extra information that a compiled language doesn't
Since computers are getting faster and faster that slow down is getting to be less and less of an issue as more people adopt interpreter based languages to solve their problems.
Since the difference between compiled and interpreted has to do with when the source code txt file is translated into machine code you might still be wondering what the difference between these languages is. What makes php so special that it can be interpreted while c++ must be compiled?
The answer is nothing. For every interpreted language it is conceivable that someone could write a compiler that will make the machine code and store it as a file before run time saving the computer from redoing that work over and over. Similarly it's conceivable that someone could write an interpreter for every compiled language. So why don't they? Well for some they have. Perl is an interpreted language that can be compiled.
When people talk about c++ being a compiled language what they mean is the traditional way to run is to compile the source to machine code and execute (run) the machine code. Even if someone has written an interpreter that can process c++ people are still going to think of c++ as a compiled language because for a long time that's all it was and is still how it is most often used. It's tradition.
How can someone use Javascript, PHP, and ASP together.
PHP and ASP are both typically used server side for web sites. Most typical reason they would be used together is because a shop started using one and decided to switch to the other. Or they really needed some third party solution that was written in the other and find themselves needing to support development in both. PHP could support one page on a site while ASP supports another.
Both could easily be working with javascript. JavaScript is run client side. Parts of it might even have been written by the server side PHP or ASP.
Or program a game in C/C++ and use python as scripts?
I've never used c/c++ and python together but I'll tell you I've used perl and java together. We use perl to automate setting up our java projects in eclipse.
Different languages are better at solving certain kinds of problems. Perl is great for smaller projects that need powerful text processing. Java is great for massive multi developer projects that need reliable ways to resolve dependencies and control complexity.
You might think two languages shouldn't be able to communicate with each other without a translator but programming languages make programs that do a job. As long is everything is doing it's job correctly and uniformly they don't actually need to talk to each other. Well, unless that is their job :)
In fact, I'd bet good money the computer you are using right now has programs running and working together that were written in more than one language.