Inferred Typing was a feature brought into the language (C# and VB) in the last couple of versions of the language in order to support anonymous typing via Linq. This is different from the old VB-Type dim
-ing that created a Variant
type.
An anonymous type is a shorthand way to pick only certain items from an object within a collection. For instance, consider the below:
dim users as new List<User>()
'...Code that adds users to the List here.
dim usernames = From U in users
Select new with { .Username = U.Username }
This takes a list of users, and creates a new Enumerable with objects that just contain the Username. It's particularly useful as it can also be used to merge lists.
Additionally, some people advocate using it when it's not important for someone reading the code to know what the object is (i.e. what concrete class it is), but simply what it represents. Jon Skeets answer gives a decent coverage of this type of thinking. It's not really what Implicit Typing was designed for, but as always, developers find uses for things the designers never considered.
It's worth noting, that Inferred Typing (and anonymous typing) is purely a Design-Time conceit. Under the hood, during compile time, the Compiler will actually create a concrete class representing the anonymous type, and will code the variable to the hard coded type. You can confirm this by looking at the IL output for the exe:
Sub Main()
Dim x = 5
Dim y As Integer = 9
End Sub
Outputs to the IL as
.locals init ([0] int32 x,
[1] int32 y)
You can see that it has initialised them as Ints. Equally, using an anonymous type:
Sub Main()
Dim data As New List(Of Integer)({1, 2, 3, 4, 5})
Dim y = From D In data
Select New With {.Val = D, .Calc = D / 100}
End Sub
Causes the compiler to create a new Type inside the exe:
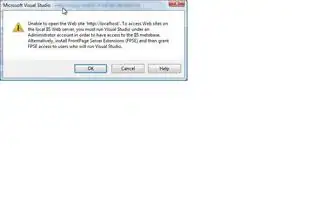
And the Code to use this type:
.locals init ([0] class [mscorlib]System.Collections.Generic.List`1<int32> data,
[1] class [mscorlib]System.Collections.Generic.IEnumerable`1<class VB$AnonymousType_0`2<int32,float64>> y,
[2] int32[] VB$LW$t_array$S0)