First of all, if you're doing something in WPF you'd better do it the WPF Way.
If you're not interested in learning XAML and MVVM, then you'd rather go back to dinosaur useless technologies.
<Window x:Class="MiscSamples.DataGridSearch"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
Title="DataGridSearch" Height="300" Width="300">
<DockPanel>
<DockPanel DockPanel.Dock="Top">
<TextBlock Text="Search:" DockPanel.Dock="Left"/>
<TextBox Text="{Binding SearchString, UpdateSourceTrigger=PropertyChanged}"/>
</DockPanel>
<DataGrid ItemsSource="{Binding ItemsView}" AutoGenerateColumns="True"/>
</DockPanel>
</Window>
Code Behind:
public partial class DataGridSearch : Window
{
public DataGridSearch()
{
InitializeComponent();
DataContext = new DataGridSearchViewModel();
}
}
ViewModel:
public class DataGridSearchViewModel: PropertyChangedBase
{
private string _searchString;
public string SearchString
{
get { return _searchString; }
set
{
_searchString = value;
OnPropertyChanged("SearchString");
ItemsView.Refresh();
}
}
private ICollectionView _itemsView;
public ICollectionView ItemsView
{
get { return _itemsView; }
}
private ObservableCollection<DataGridSearchModel> _items;
public ObservableCollection<DataGridSearchModel> Items
{
get { return _items ?? (_items = new ObservableCollection<DataGridSearchModel>()); }
}
public DataGridSearchViewModel()
{
_itemsView = CollectionViewSource.GetDefaultView(Items);
_itemsView.Filter = x => Filter(x as DataGridSearchModel);
Enumerable.Range(0, 100)
.Select(x => CreateRandomItem())
.ToList()
.ForEach(Items.Add);
}
private bool Filter(DataGridSearchModel model)
{
var searchstring = (SearchString ?? string.Empty).ToLower();
return model != null &&
((model.LastName ?? string.Empty).ToLower().Contains(searchstring) ||
(model.FirstName ?? string.Empty).ToLower().Contains(searchstring) ||
(model.Address ?? string.Empty).ToLower().Contains(searchstring));
}
private DataGridSearchModel CreateRandomItem()
{
return new DataGridSearchModel
{
LastName = RandomGenerator.GetNext(1),
FirstName = RandomGenerator.GetNext(1),
Address = RandomGenerator.GetNext(4)
};
}
}
Data Item:
public class DataGridSearchModel:PropertyChangedBase
{
public string LastName { get; set; }
public string FirstName { get; set; }
public string Address { get; set; }
}
PropertyChangedBase (MVVM helper class):
public class PropertyChangedBase:INotifyPropertyChanged
{
public event PropertyChangedEventHandler PropertyChanged;
protected virtual void OnPropertyChanged(string propertyName)
{
Application.Current.Dispatcher.BeginInvoke((Action) (() =>
{
PropertyChangedEventHandler handler = PropertyChanged;
if (handler != null) handler(this, new PropertyChangedEventArgs(propertyName));
}));
}
}
Random Generator (just to generate random strings)
public static class RandomGenerator
{
private static string TestData = "Lorem ipsum dolor sit amet, consectetur adipisicing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo consequat. Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum";
private static List<string> words;
private static int maxword;
private static Random random = new Random();
static RandomGenerator()
{
words = TestData.Split(' ').ToList();
maxword = words.Count - 1;
}
public static string GetNext(int wordcount)
{
return string.Join(" ", Enumerable.Range(0, wordcount)
.Select(x => words[random.Next(0, maxword)]));
}
public static int GetNextInt(int min, int max)
{
return random.Next(min, max);
}
}
Result:
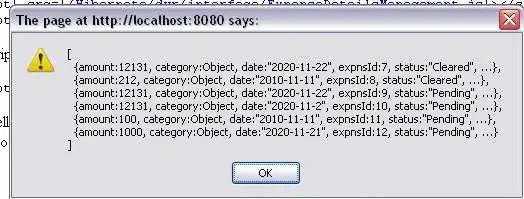
- Fully MVVM.
- There's not a single line of code that manipulates any UI element. This is the WPF way.
UpdateSourceTrigger=PropertyChanged
in the TextBox
binding makes it search as you type.
- Fully strongly typed object model much better than using
DataTables
and stuff like that.
- WPF rocks. Just copy and paste my code in a
File -> New Project -> WPF Application
and see the results for yourself.
- Forget the winforms mentality, it's irrelevant, clumsy, generates bad code and just feels noob and immature.