The following example can help to understand the differences between pointers of different types:
#include <stdio.h>
int main()
{
// Pointer to char
char * cp = "Abcdefghijk";
// Pointer to int
int * ip = (int *)cp; // To the same address
// Try address arithmetic
printf("Test of char*:\n");
printf("address %p contains data %c\n", cp, *cp);
printf("address %p contains data %c\n", (cp+1), *(cp+1));
printf("Test of int*:\n");
printf("address %p contains data %c\n", ip, *ip);
printf("address %p contains data %c\n", (ip + 1), *(ip + 1));
return 0;
}
The output is:
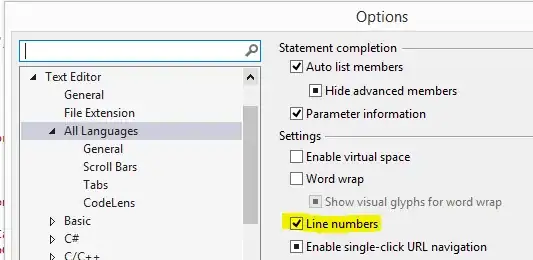
It is important to understand that address+1
expression gives different result depending on address
type, i.e. +1
means sizeof(addressed data)
, like sizeof(*address)
.
So, if in your system (for your compiler) sizeof(int)
and sizeof(char)
are different (e.g., 4 and 1), results of cp+1
and ip+1
is also different. In my system it is:
E05859(hex) - E05858(hex) = 14702684(dec) - 14702681(dec) = 1 byte for char
E0585C(hex) - E05858(hex) = 14702684(dec) - 14702680(dec) = 4 bytes for int
Note: specific address values are not important in this case. The only difference is the variable type the pointers hold, which clearly is important.
Update:
By the way, address (pointer) arithmetic is not limited by +1
or ++
, so many examples can be made, like:
int arr[] = { 1, 2, 3, 4, 5, 6 };
int *p1 = &arr[1];
int *p4 = &arr[4];
printf("Distance between %d and %d is %d\n", *p1, *p4, p4 - p1);
printf("But addresses are %p and %p have absolute difference in %d\n", p1, p4, int(p4) - int(p1));
With output:
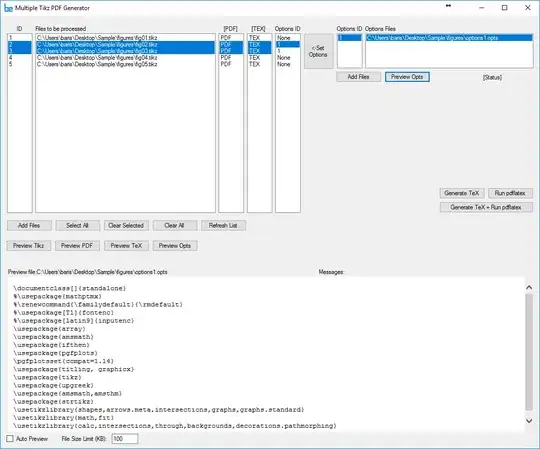
So, for better understanding, read the tutorial.