Vue.js is an open-source, progressive JavaScript framework for building user interfaces that aims to be incrementally adoptable. Vue.js is mainly used for front-end development and requires an intermediate level of HTML and CSS. Vue.js questions are highly version specific and should always be tagged with [vuejs2] or [vuejs3] in addition to this tag.
What is Vue.js?
Vue.js is an open-source, progressive JavaScript framework for building user interfaces that aims to be incrementally adoptable.
Instead of performing manual updates to the DOM, which can be repetitive and error prone (think jQuery), Vue embraces the idea of "data-driven views," where changes in data drive changes in the DOM.
This idea forms the core of Vue.js: a reactive data-binding system that is designed to make it extremely simple to keep your data and the DOM in sync.
What makes Vue particularly powerful, however, is that it can be built upon, increasing its functionality from a simple view-model library to that of a fully fledged JavaScript framework capable of powering entire SPA's via supporting plugins and libraries such as Vue Router, Vue Resource, and Vuex.
Features
Vue.js includes:
- Dead simple, unobtrusive reactivity using plain JavaScript objects.
- Component-oriented development style with tooling support
- Lean and extensible core
- Flexible transition effect system
- Fast performance out of the box, without the need for complex optimization
- Support for single file components, which allow HTML, JavaScript, and CSS in the same file
2.0 also includes:
- The option to write components using virtual DOM
- Server side rendering
- The option to use JSX with components
Vue 3.0 is currently in development, and includes some notable changes and improvements detailed here on VueJS.org.
Questions that are version specific should be tagged with vuejs2 or vuejs3 respectively.
Vue is compatible with all ES5-compliant browsers. As such, IE8 and below are unsupported.
To check out an in-depth guide with live examples or the official docs, visit vuejs.org.
Code Example
All that is required to use Vue in a project is to create a Vue instance, and tell it where to render in the DOM. Vue handles the rest!
The code below forms a simple, fully functioning Vue app:
<html>
<div id="demo">
<p>{{ message }}</p>
<input v-model="message">
</div>
<script src="https://unpkg.com/vue@2"></script>
<script>
new Vue({
el: '#demo', //Tells Vue to render in HTML element with id "demo"
data() {
return {
message: 'Hello Vue.js!'
}
}
});
</script>
</html>
See a live and editable version here.
Related Tags
UI Frameworks built with Vue.js
Tooling
- Vite (Standard Tooling for Vue.js Development)
- Debugging Vue.js applications. (Browser devtools extension)
Resources
- Official Website
- Official Vue.js Guide - A great place to start diving in!
- Awesome Vue
- Vue Curated - Find the best packages for your Vue app!
- Vue Forum
- Vue on Discord
- The Vue Point (official blog)
- Roadmap
- VueJobs
- Vue.js on twitter
- Vue Style Guide
- Comparison of Vue to other frameworks
Official Logo:
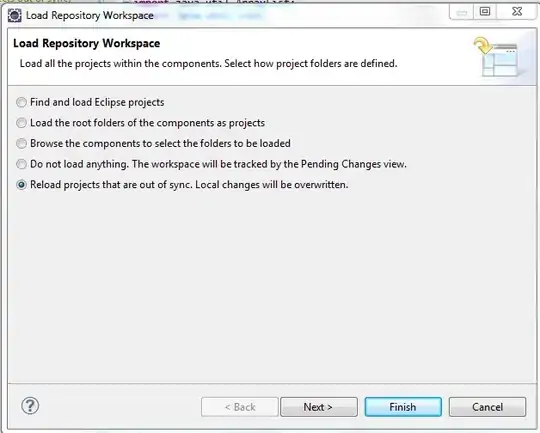
Using Vue in Stack Snippets
It is often useful to share runnable snippets of code in questions regarding Vue components. Since Stack Snippets can't natively run Vue single file components (.vue
files), it's necessary to do a little conversion work.
To create a Stack Snippet containing Vue in a Stack Overflow post, make sure you:
- Import Vue into the HTML section of the snippet
- Wrap component JavaScript with
new Vue({...})
- Replace
default export
withnew Vue
, and don't forget the parenthesis()
around your brackets{}
- Note that you won't be able to use
import
statements, so you should trim everything out of the snippet that's not strictly necessary to understand your question and the problem at hand - You may also need to add example data if your component relied on props or APIs
- Replace
- Tell the new Vue instance where to render in your HTML
- Use the
el
property of the new Vue instance to indicate what HTML container Vue should render in (see example below)
- Use the
You may also want to replace your <template>
HTML elements with some alternative (ie. <div>
) if it causes issues.
Starting with something like this:
HelloWorld.vue
<template>
<div>
<p>{{ message }}</p>
<input v-model="message">
</div>
</template>
<script>
export default {
data() {
return {
message: 'Hello World!'
}
}
}
</script>
Will end up with something like this:
HelloWorld.html
<html>
<div id="app">
<p>{{ message }}</p>
<input v-model="message">
</div>
<!-- Don't forget to include Vue from CDN! -->
<script src="https://unpkg.com/vue@2"></script>
<script>
new Vue({
el: '#app', //Tells Vue to render in HTML element with id "app"
data() {
return {
message: 'Hello World!'
}
}
});
</script>
</html>
You can drop this straight into the HTML section of a snippet, or split it into the HTML and JavaScript sections accordingly. Bear in mind that splitting it may make the code clearer to readers!